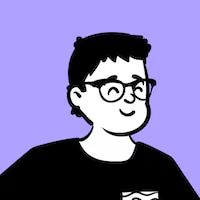
C++
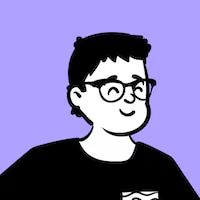
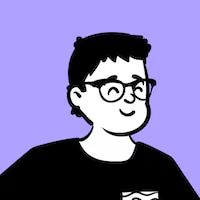
Mutexes - Locking methods in C++ 👋
In C++, a mutex (short for mutual exclusion) is a synchronization primitive used to protect shared resources from simultaneous access by multiple threads. It ensures that only one thread can access the protected resource at a given time, preventing data races and maintaining thread safety.
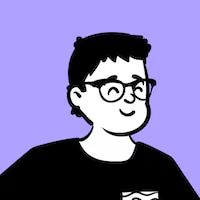
How to pass reference to a lambda in C++ 👋
A lambda function is an anonymous function that can be defined inline without a separate function declaration. It allows you to create small, local functions with captured variables and can be used in situations where a function object or function pointer is expected. Lambdas are especially useful in scenarios where you need to pass a function as an argument or when you want to define a function inside another function.
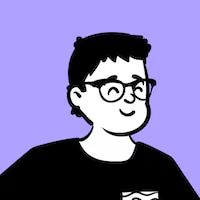
Polymorphism in C++ 👋
Polymorphism means many forms. Ability of a message to be displayed in many forms is what it is all about. Polymorphism is very important feature in Object Oriented Programming. In C++, we have four different types of polymorphism as mentioned below
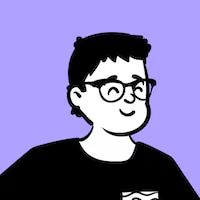
A Taxonomy of Expression Value Categories in C++👋
C++ had value categories in C++0X version. The two value categories that were before C++11 are lvalues and rvalues. One of the greatest addition to C++11 was the introduction of movable types. Learning about the value categories is one of the pre-requisites to learn about move semantics. Any expression before the C++11 standard was either lvalue or rvalue. lvalue has an identity and its lifetime depends on the scope of the variable. On the other hand, rvalues don’t have an identity and it does not live beyond the life of the expression.
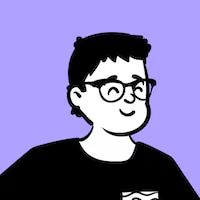
Using Templates for Generic Programming - Part 1 (Embracing the beauty of SFINAE and enable_if)👋
In this blog post, we will learn advanced template programming concepts. These concepts include how to change the implementation of classes and functions based on the type provided, how to work with different arguments and how to properly forward them, how to optimize the code in both runtime and compile-time, about SFINAE, std::enable_if
, std::enable_if_t
.
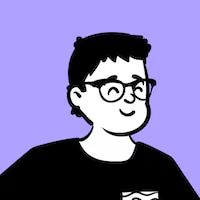
C++ Best Practice #1: Don't simply use: using namespace std; 👋
Never try to import the whole std namespace into your program. Namespaces are developed to avoid naming conflicts. But, when we import the whole namespace there is a possibility that this could lead to name conflicts. You can do more harm than more good.