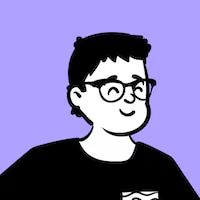
C++
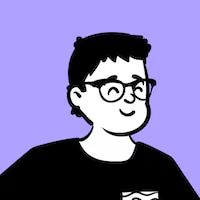
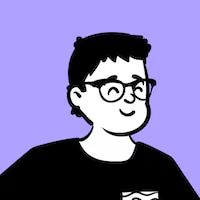
Beautiful code #7 - std::thread, std::accumulate π
Here is an example of C++ threading code that demonstrates how to use multiple threads to calculate the sum of an array of numbers. I will provide a detailed explanation of the code afterwards.
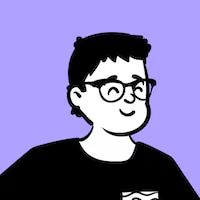
What is new in <numeric> library in C++17? π
The
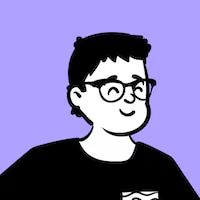
Beautiful code #6 - std::copy_if, std::remove_copy_if, std::copy, std::execution::par_unseq, std::back_inserter π
std::copy_if
, std::remove_copy_if
, std::copy
, std::back_inserter
, and std::execution::par_unseq
are features from C++11 and C++98 that helps efficiently copy from one data structure to the another.
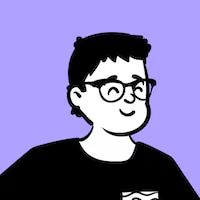
Beautiful code #5 - std::for_each, std::ranges::for_each, std::optional, std::execution::par_unseq, mutable π
std::for_each
, std::ranges::for_each
, std::optional
, and std::execution::par_unseq
are features from C++20 and C++23 that helps efficiently loop through the loop, loop in parallel, add optional values in the data structure of your choice, etc.
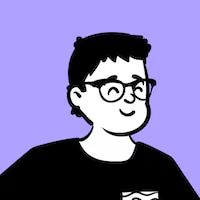
Beautiful code #4 - std::any_of, std::none_of, std::all_of - efficient C++20 featureπ
std::any_of
, std::none_of
and std::all_of
is a C++20 feature that helps efficiently check for basic conditions in the data structures. This is very optimal because of early termination once some fact is found and the algorithm don’t have to iterate more to further prove anything before returning the result.
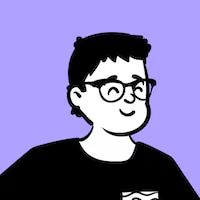
Beautiful code #3 - [[no_unique_address]] - sophisticated and simple C++20 featureπ
[[no_unique_address]]
is a C++ 20 feature that helps in saving memory while declaring an empty struct or class and creating object for it in some other place.