Quick summary ↬ std::for_each, std::ranges::for_each, std::optional, and std::execution::par_unseq are features from C++20 and C++23 that helps efficiently loop through the loop, loop in parallel, add optional values in the data structure of your choice, etc.
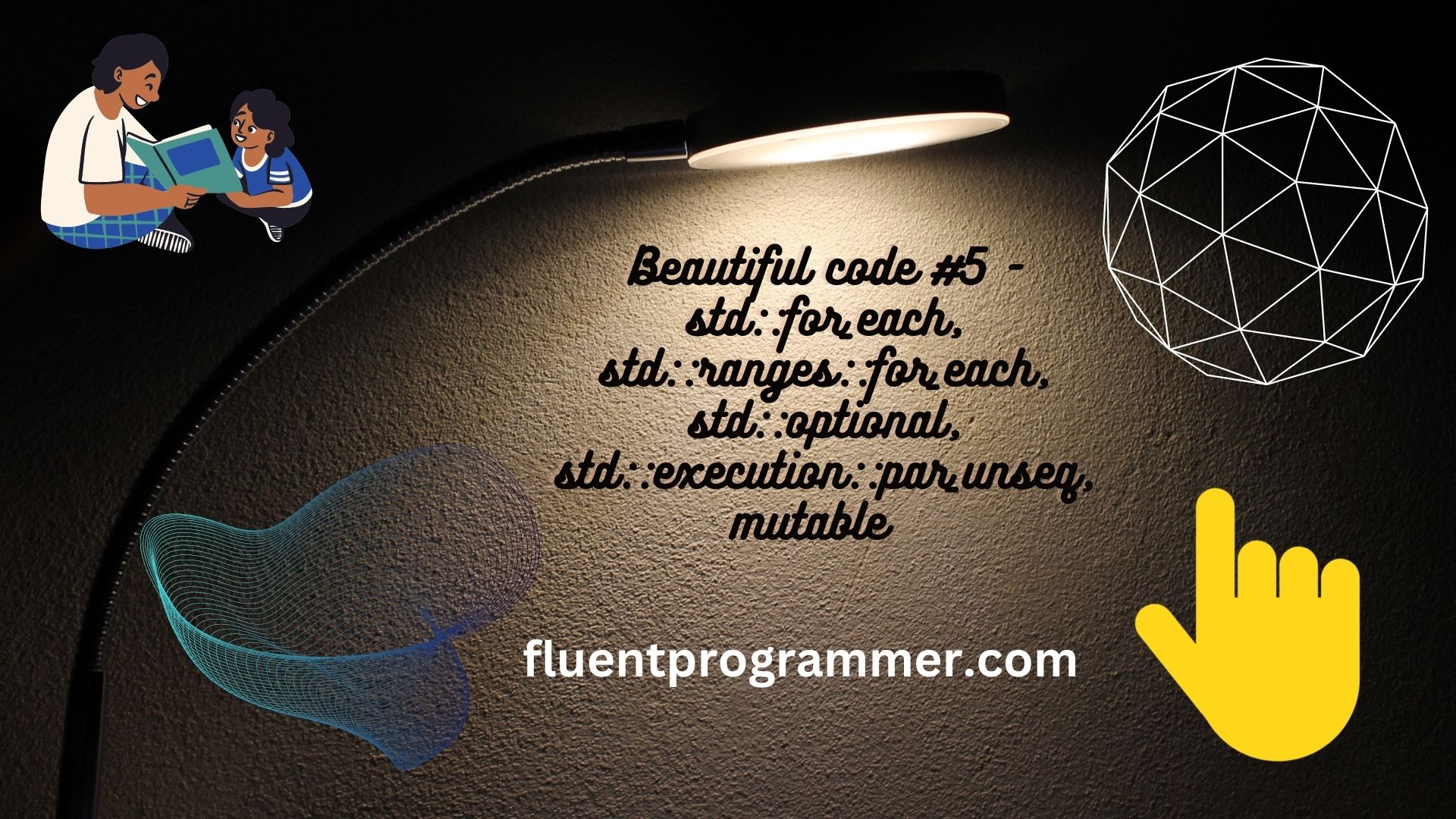
Beautiful code #5
|
|
Beautiful code #5 Explanation
The above C++ code snippet make use of all the four functions std::for_each
, std::ranges::for_each
, std::optional
, std::execution::par_unseq
, and mutable
.
std::for_each
as used in line number 12
accepts three arguments the first one an iterator that represents the beginning of an iterator, the second one an iterator that represents the end of an iteration and the third is a simple lambda function.
|
|
Curious about the mutable
keyword?? It is used to make sure the value of i
if updated in the loop will be maintained that way.
All the three arguments were already explained and hope it is clear.
|
|
22
to 25
is another version of the same for loop but it only works in the C++17 and versions after that since std::execution is new and introduced in C++17. The feature was proposed by Pablo Halpern and Michael Garland and was accepted as part of the standardization effort. Argument 1
is std::execution::par_unseq
which is to mention that this for loop can be executed in parallel and in unsequenced manner. The second and third argument is about the starting and ending iterators and the fourth argument is a method name process
that is called for every value of the vector.
Now look at the line number 37
to 49
- it has an another variant of for_each
using the ranges
library. The first argument is the actual data structure, the second argument is a lambda function and the third one is the projection lambda function. Always the projection lambda function runs first to see whether the std::vector
that stores the std::optional<int>
has value or not. value_or
is a method to check whether std::optional
has the value or not and assign a default value if it does not has a value.
The the actual lambda function will run and print the value one by one from the std::vector
.
Conclusion
I covered std::for_each
, std::ranges::for_each
, mutable
, std::optional
, std::execution::par_unseq
. Hope you enjoyed it.
You will also like — More Articles
https://fluentprogrammer.com/beautiful-code-1-using-define-templates-r-value-reference/ https://fluentprogrammer.com/beautiful-code-2-enable_if_t-template-inside-a-template/ https://fluentprogrammer.com/beautiful-code-3-no_unique_address_cpp_20-feature/ http://fluentprogrammer.com/beautiful-code-4-any_of-none_of-all_of/ https://fluentprogrammer.com/beautiful-code-6-copy_if-remove_copy_if-copy-back_inserter/