Quick summary ↬ std::any_of, std::none_of and std::all_of is a C++20 feature that helps efficiently check for basic conditions in the data structures. This is very optimal because of early termination once some fact is found and the algorithm don’t have to iterate more to further prove anything before returning the result.
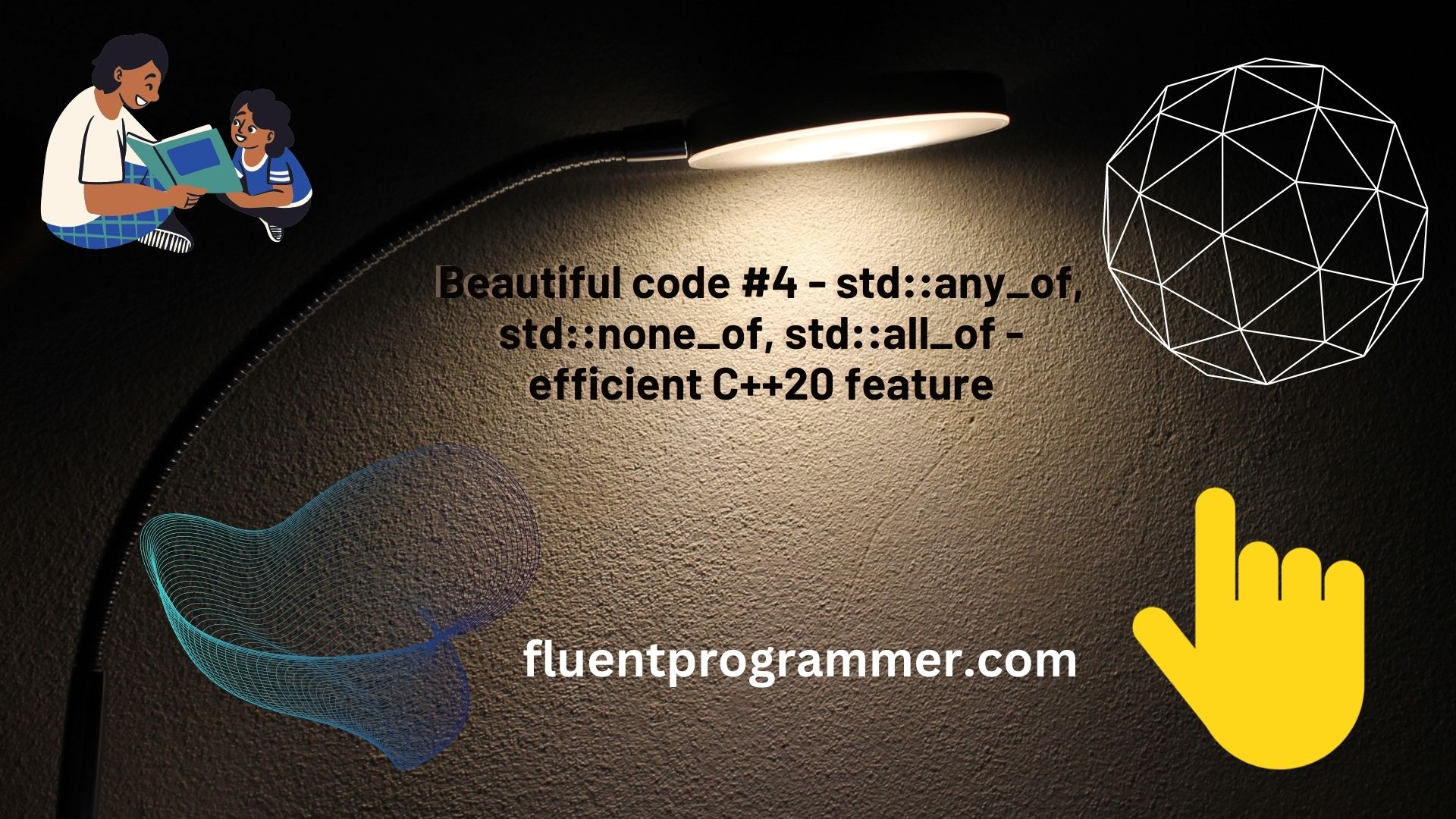
Beautiful code #4
|
|
Beautiful code #4 Explanation
The above C++ code snippet make use of all the three functions std::any_of
, std::none_of
, std::none_of
.
std::all_of
std::all_of
as used in bool output_1 = std::all_of(data.begin(), data.end(), is_even);
iterates through the vector data
until it find an evidence to return a boolean false
. In the above std::all_of
code, the third argument is a lambda function that returns true
whenever the value is even. If it finds and odd the std::all_of
does an early termination and returns false
.
If we pass an empty vector then the std::all_of
returns false
.
|
|
The above code proves that std::all_of
has early termination in place.
std::none_of
std::none_of
as used in bool output_2 = std::none_of(data.begin(), data.end(), is_odd);
iterates through the vector data
to make sure none of the value is an odd. If any value is odd then the function std::none_of
returns false
else true
.
If we pass an empty vector then the std::none_of
returns true
by default..
std::any_of
std::any_of
as used in bool output_3 = std::any_of(data.begin(), data.end(), is_double_digit);
iterates through the vector and check for any double digit value. If it find any that met the condition then it returns true
else false
.
If we pass an empty vector then the std::any_of
returns false
by default.
Conclusion
All these algorithms has worst case runtime of O(n) and I find it beautiful. Hope you enjoyed it !!
You will also like — More Articles
https://fluentprogrammer.com/beautiful-code-1-using-define-templates-r-value-reference/ https://fluentprogrammer.com/beautiful-code-2-enable_if_t-template-inside-a-template/ https://fluentprogrammer.com/beautiful-code-3-no_unique_address_cpp_20-feature/ https://fluentprogrammer.com/beautiful-code-5-for_each-optional/ https://fluentprogrammer.com/beautiful-code-6-copy_if-remove_copy_if-copy-back_inserter/